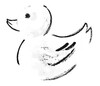 |
Darwin
1.0
Event loop based prototype framework
|
#include <boost/test/included/unit_test.hpp>
#include <boost/exception/all.hpp>
#include "test.h"
#include "exceptions.h"
#include "Objects.h"
#include <limits>
#include <iostream>
|
static const auto | feps = numeric_limits<float>::epsilon() |
|
◆ BOOST_TEST_MODULE
#define BOOST_TEST_MODULE testObjects |
◆ BOOST_AUTO_TEST_CASE() [1/4]
BOOST_AUTO_TEST_CASE |
( |
dijets |
| ) |
|
29 BOOST_TEST( std::abs(jet1.
area - 2*M_PI*0.4) <
feps );
32 jet1.
scales = {1.1, 0.5, 1.7};
33 jet2.
scales = {1.1, 0.5, 1.7};
39 BOOST_TEST( jet1.
CorrPt() == 42*0.5 );
40 BOOST_TEST( jet2.
CorrPt() == 24*0.5 );
43 BOOST_REQUIRE_NO_THROW( jet1 + jet2 );
45 BOOST_TEST( dijet.Pt() == 42 + 24 );
47 BOOST_TEST( dijet.
scales.at(1) == 0.5 );
48 BOOST_TEST( dijet.
CorrPt() == (42+24)*0.5 );
49 BOOST_TEST( dijet.
weight() == jet1.
weight() * jet2.weight() );
53 cout << jet1 <<
'\t' << jet2 <<
'\t' << dijet << endl;
56 BOOST_TEST( jet1.Pt() == 0 );
57 BOOST_TEST( jet1.
pdgID == 0 );
60 BOOST_TEST( std::abs(jet1.
weights.front() - 1) <
feps );
61 BOOST_TEST( jet1.
weights.size () == (
size_t) 1 );
62 BOOST_TEST( std::abs(jet1.
scales.front() - 1) <
feps );
63 BOOST_TEST( jet1.
scales.size () == (
size_t) 1 );
64 #if BOOST_VERSION < 107600
65 BOOST_REQUIRE_THROW( jet1 + jet2, boost::wrapexcept<length_error> );
66 #endif // TODO: fix... (note sure that the version is the problem)
◆ BOOST_AUTO_TEST_CASE() [2/4]
BOOST_AUTO_TEST_CASE |
( |
genevents |
| ) |
|
72 BOOST_TEST( std::abs(event.
weights.front() - 1) <
feps );
73 BOOST_TEST( event.
weights.size () == (
size_t) 1 );
75 BOOST_TEST( event.
process == 0. );
77 event.weights.front() = 42;
78 event.weights.push_back(24);
81 BOOST_TEST( std::abs(event.
weight() - 24) <
feps );
84 BOOST_TEST( std::abs(event.
weights.front() - 1) <
feps );
85 BOOST_TEST( event.
weights.size () == (
size_t) 1 );
87 BOOST_TEST( event.
process == 0. );
89 cout <<
event << endl;
◆ BOOST_AUTO_TEST_CASE() [3/4]
BOOST_AUTO_TEST_CASE |
( |
pileup |
| ) |
|
127 BOOST_TEST( pileup.
GetTrPU() == 42 );
128 BOOST_TEST( pileup.
GetTrPU(
'+') > 42 );
129 BOOST_TEST( pileup.
GetTrPU(
'-') < 42 );
130 BOOST_TEST( pileup.
GetIntPU() == 24 );
131 BOOST_TEST( pileup.
GetIntPU(
'+') > 24 );
132 BOOST_TEST( pileup.
GetIntPU(
'-') < 24 );
134 TRandom3 r1(23413), r2(45346);
138 BOOST_TEST( pileup.
rho == 0 );
139 BOOST_TEST( pileup.
nVtx == 0 );
140 BOOST_TEST( pileup.
trpu == 0 );
141 BOOST_TEST( pileup.
intpu == 0 );
◆ BOOST_AUTO_TEST_CASE() [4/4]
BOOST_AUTO_TEST_CASE |
( |
recevents |
| ) |
|
95 BOOST_TEST( std::abs(event.
weights.front() - 1) <
feps );
96 BOOST_TEST( event.
weights.size () == (
size_t) 1 );
97 BOOST_TEST( event.
fill == 0 );
98 BOOST_TEST( event.
runNo == 0 );
99 BOOST_TEST( event.
lumi == 0 );
100 BOOST_TEST( event.
evtNo == (
unsigned long long) 0 );
102 event.weights.front() = 42;
103 event.weights.push_back(24);
106 BOOST_TEST( std::abs(event.
weight() - 24) <
feps );
109 BOOST_TEST( std::abs(event.
weights.front() - 1) <
feps );
110 BOOST_TEST( event.
weights.size () == (
size_t) 1 );
111 BOOST_TEST( event.
fill == 0 );
112 BOOST_TEST( event.
runNo == 0 );
113 BOOST_TEST( event.
lumi == 0 );
114 BOOST_TEST( event.
evtNo == (
unsigned long long) 0 );
116 cout <<
event << endl;
◆ feps
const auto feps = numeric_limits<float>::epsilon() |
|
static |
float hard_scale
hard scale, corresponding to pthat in Pythia 8
Definition: Objects.h:29
static size_t iWgt
global index for weight
Definition: Objects.h:26
unsigned long long evtNo
event number
Definition: Objects.h:46
float rho
soft activity (see formula 7.15 in Patrick's thesis)
Definition: Objects.h:101
static size_t iScl
global index for scale
Definition: Objects.h:192
int process
flag for process (TODO?)
Definition: Objects.h:28
int runNo
6-digit run number
Definition: Objects.h:44
float weight() const final
uses iWgt internally
Definition: Objects.cc:22
static float R
parameter of clustering algorithm
Definition: Objects.h:147
void clear()
resets all members to the same values as in the constructor
Definition: Objects.cc:113
static const auto feps
Definition: Objects.cc:19
char pdgID
pdgID (default is 0 = undefined)
Definition: Objects.h:149
float weight() const final
uses iWgt internally
Definition: Objects.cc:240
float weight() const final
uses iWgt internally
Definition: Objects.cc:37
float trpu
true pile-up
Definition: Objects.h:103
float GetTrPU(const char='0') const
true pile-up variations
Definition: Objects.cc:87
int lumi
lumi section
Definition: Objects.h:45
std::vector< float > weights
jet weight (typically expected to be trivial for all-flavour inclusive jet cross section)
Definition: Objects.h:155
std::vector< float > weights
Definition: Objects.h:16
static size_t iWgt
global index for weight
Definition: Objects.h:41
float GetIntPU(const char='0') const
in-time pile-up variations
Definition: Objects.cc:97
int nVtx
number of vertices in the event
Definition: Objects.h:102
char nCHadrons
number of C hadrons (0, 1+ in 2017; 0, 1, 2+ in 2016)
Definition: Objects.h:151
float area
jet area, roughly = pi*R^2
Definition: Objects.h:200
std::vector< float > scales
Definition: Objects.h:154
char nBHadrons
number of B hadrons (0, 1 or 2+)
Definition: Objects.h:150
static float MBxsec
minimum-bias cross section
Definition: Objects.h:98
int intpu
in-time pile-up (i.e. from the same bunch crossing)
Definition: Objects.h:104
static float MBxsecRelUnc
relative uncertainty on minimum-bias cross section
Definition: Objects.h:99
Structure for events in MC only with generator information.
Definition: Objects.h:25
float CorrPt() const final
uses iScl internally
Definition: Objects.cc:241
A detector level jet.
Definition: Objects.h:189
void clear() override
resets all members to the same values as in the constructor
Definition: Objects.cc:246
float pthatMax
hard-scale of the hardest pile-up event (to deal with anomalous events)
Definition: Objects.h:105
int fill
4-digit LHC fill
Definition: Objects.h:43